In this section, we'll show you how to use FusionCharts and Ruby to plot charts from data contained in a database. We'll create a pie chart to show "Factory Output Quantity by Factory" using dataXML method.
For the sake of ease, we'll use the MySQL Database. You can, however, use any database with FusionCharts including SQLLite, MS SQL, Oracle, Access etc. Database configuration will be available here Download Package >> RoR >> config >> database.yml. In the production version, we have used database named as factorydb.
Before you go further with this page, we recommend you to please see the previous section "Basic Examples" as we start off from concepts explained in that page.
All code discussed here is present in
Controller : Download Package > Code > RoR > SampleApp > app > controllers > fusioncharts > db_example_controller.rb.
Rhtml : Download Package > Code > RoR > SampleApp > app > views > fusioncharts > db_example folder.
Let's quickly have a look at the database structure. 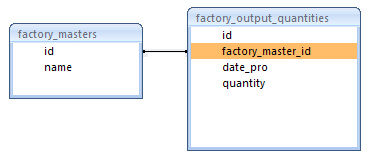 The database contains just 2 tables:
- factory_masters: To store the name and id of each factory
- factory_output_quantities: To store the number of units produced by each factory for a given date.
Note that the name of the table is pluralized, as per Ruby conventions. For demonstration, we've fed some sample data in the database.
- All the database configuration is present in the file app > config > database.yml. Here we need to specify the database name, user name and password to access the database. We have used the MySQL database for our examples. We assume that you have created the database with the name factorydb, either by using the rake db:create utility or by using MySQL create database command.
- Once this is done, we need to create the required tables. The required sql script "create_tables.sql" is present in the Download Package > Code > RoR >db folder. You could run this script in your mysql and create the tables and insert the sample data. The other way of creating these tables, is to run rake db:migrate for the migration scripts "001_create_factory_masters.rb" and "002_create_factory_output_quantities.rb" present in the folder Download Package > Code > RoR >db > migrate. Note that these scripts will not create foreign key relationships. You would have to manually alter the table to create these relationships, if you think neccessary. To insert the sample data please run the sql script "insert_sample_data.sql" present in the Download Package > Code > RoR >db folder.
Let's now shift our attention to the code that will interact with the database, fetch data and then render a chart.
Controller: Fusioncharts::DbExampleController
Action: basic_dbexample
class Fusioncharts::DbExampleController < ApplicationController
layout "common"
def basic_dbexample
response.content_type = Mime::HTML
@factories = Fusioncharts::FactoryMaster.find(:all)
end
View:
<% @page_title="FusionCharts - Database Example" %>
<% @page_heading="FusionCharts Database Example Using dataXML method" %>
<% @page_subheading="Click on any pie slice to slice it out. Or, right click to enable rotation mode." %>
<%
str_xml = render :file=>"fusioncharts/db_example/basic_factories_quantity",:locals=>{:factories => @factories}
render_chart '/FusionCharts/Pie3D.swf', '', str_xml, 'FactorySum', 600, 300, false, false do-%>
<% end-%>
Now, here we need to understand some lines of code.
- The basic_dbexample action of the controller, first preforms a find on FactoryMaster model, getting all the values.
- For each factory in the record set obtained in the previous step, we need the total output quantity for that factory. This is done in the FactoryMaster Model. Note that FactoryMaster and FactoryOutputQuantity are related to each other as shown:
class Fusioncharts::FactoryMaster < ActiveRecord::Base
has_many :factory_output_quantities,
:order => 'date_pro asc'
def total_quantity
self.factory_output_quantities.sum(:quantity)
end
end
class Fusioncharts::FactoryOutputQuantity < ActiveRecord::Base
belongs_to :factory_master
end
Based on this relation, Ruby On Rails is able to get the data for FactoryOutputQuantity when a find is performed on FactoryMaster.
- The view basic_dbexample.html.erb passes the @factories present in the controller to the builder file "basic_factories_quantity", as locals parameter. The xml obtained from the builder is assigned to str_xml variable.
- Finally, render_chart function is called by setting the str_xml as xml parameter.
Let us now take a look at the builder file. Builder - basic_factories_quantity.builderxml = Builder::XmlMarkup.new
xml.chart(:caption=>'Factory Output report', :subCaption=>'By Quantity', :pieSliceDepth=>'30', :showBorder=>'1', :formatNumberScale=>'0', :numberSuffix=>'Units') do
factories.each do |factory|
xml.set(:label=>factory.name,:value=>factory.total_quantity)
end
end
The builder builds chart element with several attributes, then iterates through the factories (received via :locals from the view) to create the <set> element with value for label as factory name and value for value attribute as total factory output.
When you now run the code, you'll see a beautiful pie chart as under:
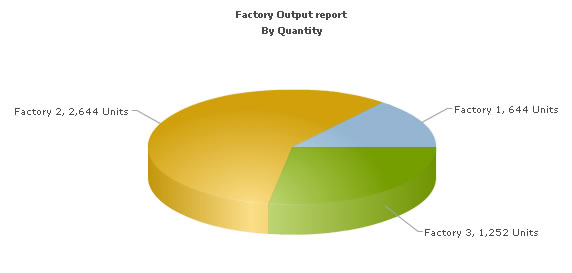
|